🚀 Introducing ejabberd 24.02: A Huge Release!
ejabberd 24.02 has just been release and well, this is a huge release with 200 commits and more in the libraries. We’ve packed this update with a plethora of new features, significant improvements, and essential bug fixes, all designed to supercharge your messaging infrastructure.
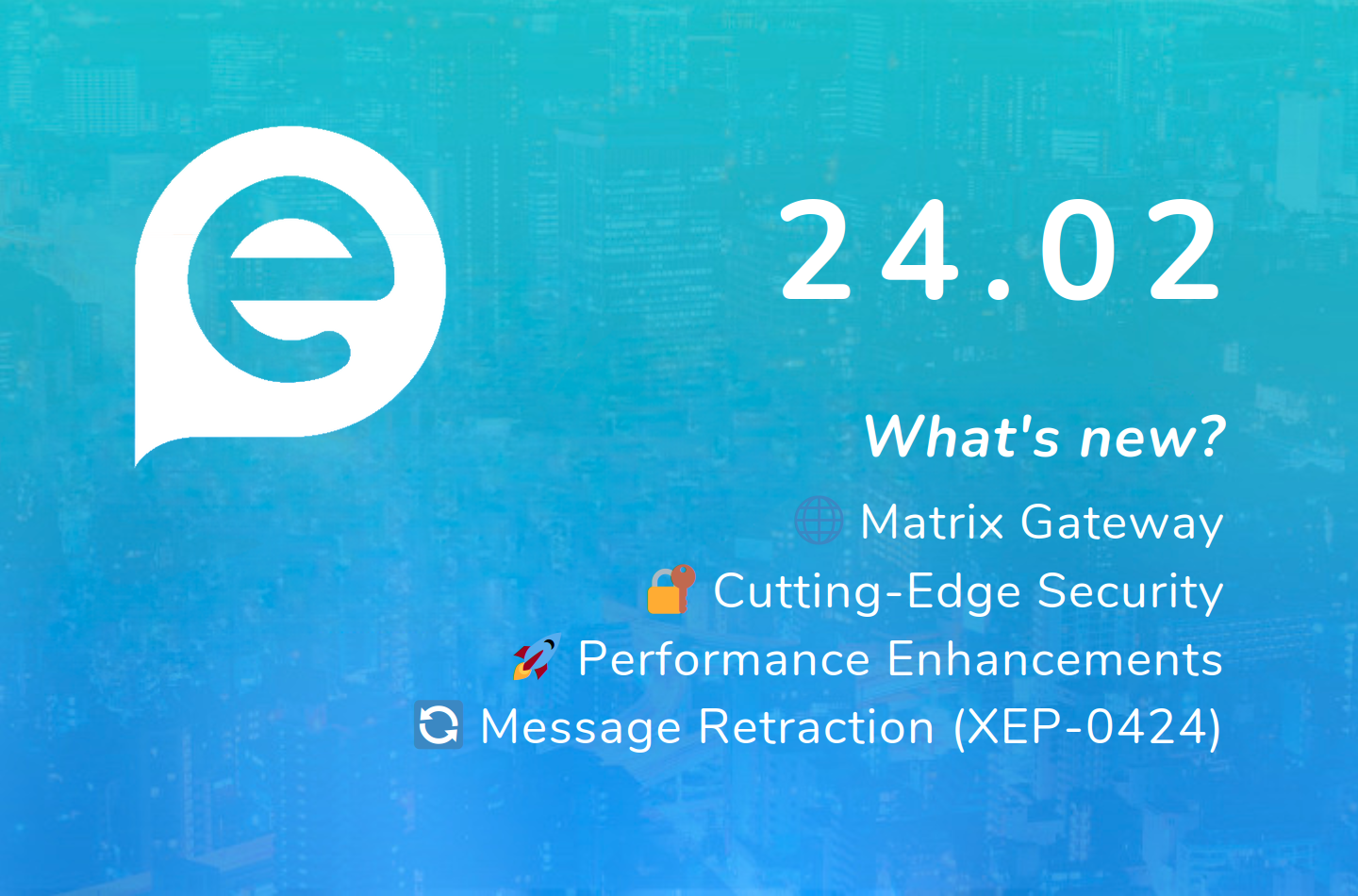
– 🌐 Matrix Federation Unleashed: Imagine seamlessly connecting with Matrix servers – it’s now possible! ejabberd breaks new ground in cross-platform communication, fostering a more interconnected messaging universe. We have still some ground to cover and for that we are waiting for your feedback.
– 🔐 Cutting-Edge Security with TLS 1.3 & SASL2: In an era where security is paramount, ejabberd steps up its game. With support for TLS 1.3 and advanced SASL2 protocols, we increase the overall security for all platform users.
– 🚀 Performance Enhancements with Bind 2: Faster connection times, especially crucial for mobile network users, thanks to Bind 2 and other performance optimizations.
– 🔄 User gains better control over on their messages: The new support for XEP-0424: Message Retraction allows users to manage their message history and remove something they posted by mistake.
– 🔧 Optimized server pings by relying on an existing mechanism coming from XEP-0198
– 📈 Streamlined API Versioning: Our refined API versioning means smoother, more flexible integration for your applications.
– 🧩 Enhanced Elixir, Mix and Rebar3 Support
If you upgrade ejabberd from a previous release, please review those changes:
A more detailed explanation of those topics and other features:
Matrix federation
ejabberd is now able to federate with Matrix servers. Detailed instructions to setup Matrix federation with ejabberd will be detailed in another post.
Here is a quick summary of the configuration steps:
First, s2s must be enabled on ejabberd. Then define a listener that uses mod_matrix_gw
:
listen:
-
port: 8448
module: ejabberd_http
tls: true
certfile: "/opt/ejabberd/conf/server.pem"
request_handlers:
"/_matrix": mod_matrix_gw
And add mod_matrix_gw
in your modules:
modules:
mod_matrix_gw:
matrix_domain: "domain.com"
key_name: "somename"
key: "yourkeyinbase64"
Support TLS 1.3, Bind 2, SASL2
Support for XEP-0424 Message Retraction
With the new support for XEP-0424: Message Retraction, users of MAM message archiving can control their message archiving, with the ability to ask for deletion.
Support for XEP-0198 pings
If stream management is enabled, let mod_ping trigger XEP-0198 <r/>equests
rather than sending XEP-0199 pings. This avoids the overhead of the ping IQ stanzas, which, if stream management is enabled, are accompanied by XEP-0198 elements anyway.
Update the SQL schema
The table archive
has a text column named origin_id
(see commit 975681). You have two methods to update the SQL schema of your existing database:
If using MySQL or PosgreSQL, you can enable the option update_sql_schema
and ejabberd will take care to update the SQL schema when needed: add in your ejabberd configuration file the line update_sql_schema: true
If you are using other database, or prefer to update manually the SQL schema:
ALTER TABLE archive ADD COLUMN origin_id text NOT NULL DEFAULT '';
ALTER TABLE archive ALTER COLUMN origin_id DROP DEFAULT;
CREATE INDEX i_archive_username_origin_id USING BTREE ON archive(username(191), origin_id(191));
ALTER TABLE archive ADD COLUMN origin_id text NOT NULL DEFAULT '';
ALTER TABLE archive ALTER COLUMN origin_id DROP DEFAULT;
CREATE INDEX i_archive_sh_username_origin_id USING BTREE ON archive(server_host(191), username(191), origin_id(191));
- PostgreSQL default schema:
ALTER TABLE archive ADD COLUMN origin_id text NOT NULL DEFAULT '';
ALTER TABLE archive ALTER COLUMN origin_id DROP DEFAULT;
CREATE INDEX i_archive_username_origin_id ON archive USING btree (username, origin_id);
ALTER TABLE archive ADD COLUMN origin_id text NOT NULL DEFAULT '';
ALTER TABLE archive ALTER COLUMN origin_id DROP DEFAULT;
CREATE INDEX i_archive_sh_username_origin_id ON archive USING btree (server_host, username, origin_id);
ALTER TABLE [dbo].[archive] ADD [origin_id] VARCHAR (250) NOT NULL;
CREATE INDEX [archive_username_origin_id] ON [archive] (username, origin_id)
WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON);
ALTER TABLE [dbo].[archive] ADD [origin_id] VARCHAR (250) NOT NULL;
CREATE INDEX [archive_sh_username_origin_id] ON [archive] (server_host, username, origin_id)
WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON);
ALTER TABLE archive ADD COLUMN origin_id text NOT NULL DEFAULT '';
CREATE INDEX i_archive_username_origin_id ON archive (username, origin_id);
ALTER TABLE archive ADD COLUMN origin_id text NOT NULL DEFAULT '';
CREATE INDEX i_archive_sh_username_origin_id ON archive (server_host, username, origin_id);
Authentication workaround for Converse.js and Strophe.js
This ejabberd release includes support for XEP-0474: SASL SCRAM Downgrade Protection, and some clients may not support it correctly yet.
If you are using Converse.js 10.1.6 or older, Movim 0.23 Kojima or older, or any other client based in Strophe.js v1.6.2 or older, you may notice that they cannot authenticate correctly to ejabberd.
To solve that problem, either update to newer versions of those programs (if they exist), or you can enable temporarily the option disable_sasl_scram_downgrade_protection
in the ejabberd configuration file ejabberd.yml
like this:
disable_sasl_scram_downgrade_protection: true
Support for API versioning
Until now, when a new ejabberd release changed some API command (an argument renamed, a result in a different format…), then you had to update your API client to the new API at the same time that you updated ejabberd.
Now the ejabberd API commands can have different versions, by default the most recent one is used, and the API client can specify the API version it supports.
In fact, this feature was implemented seven years ago, included in ejabberd 16.04, documented in ejabberd Docs: API Versioning… but it was never actually used!
This ejabberd release includes many fixes to get API versioning up to date, and it starts being used by several commands.
Let’s say that ejabberd 23.10 implemented API version 0, and this ejabberd 24.02 adds API version 1. You may want to update your API client to use the new API version 1… or you can continue using API version 0 and delay API update a few weeks or months.
To continue using API version 0:
– if using ejabberdctl, use the switch --version 0
. For example: ejabberdctl --version 0 get_roster admin localhost
– if using mod_http_api, in ejabberd configuration file add v0
to the request_handlers
path. For example: /api/v0: mod_http_api
Check the details in ejabberd Docs: API Versioning.
ejabberd commands API version 1
When you want to update your API client to support ejabberd API version 1, those are the changes to take into account:
– Commands with list arguments
– mod_http_api does not name integer and string results
– ejabberdctl with list arguments
– ejabberdctl list results
All those changes are described in the next sections.
Commands with list arguments
Several commands now use list
argument instead of a string
with separators (different commands used different separators: ;
:
\\n
,
).
The commands improved in API version 1:
– add_rosteritem
– oauth_issue_token
– send_direct_invitation
– srg_create
– subscribe_room
– subscribe_room_many
For example, srg_create
in API version 0 took as arguments:
{"group": "group3",
"host": "myserver.com",
"label": "Group3",
"description": "Third group",
"display": "group1\\ngroup2"}
now in API version 1 the command expects as arguments:
{"group": "group3",
"host": "myserver.com",
"label": "Group3",
"description": "Third group",
"display": ["group1", "group2"]}
mod_http_api not named results
There was an incoherence in mod_http_api results when they were integer/string and when they were list/tuple/rescode…: the result contained the name, for example:
$ curl -k -X POST -H "Content-type: application/json" -d '{}' "http://localhost:5280/api/get_loglevel/v0"
{"levelatom":"info"}
Staring in API version 1, when result is an integer or a string, it will not contain the result name. This is now coherent with the other result formats (list, tuple, …) which don’t contain the result name either.
Some examples with API version 0 and API version 1:
$ curl -k -X POST -H "Content-type: application/json" -d '{}' "http://localhost:5280/api/get_loglevel/v0"
{"levelatom":"info"}
$ curl -k -X POST -H "Content-type: application/json" -d '{}' "http://localhost:5280/api/get_loglevel"
"info"
$ curl -k -X POST -H "Content-type: application/json" -d '{"name": "registeredusers"}' "http://localhost:5280/api/stats/v0"
{"stat":2}
$ curl -k -X POST -H "Content-type: application/json" -d '{"name": "registeredusers"}' "http://localhost:5280/api/stats"
2
$ curl -k -X POST -H "Content-type: application/json" -d '{"host": "localhost"}' "http://localhost:5280/api/registered_users/v0"
["admin","user1"]
$ curl -k -X POST -H "Content-type: application/json" -d '{"host": "localhost"}' "http://localhost:5280/api/registered_users"
["admin","user1"]
ejabberdctl with list arguments
ejabberdctl now supports list and tuple arguments, like mod_http_api and ejabberd_xmlrpc. This allows ejabberdctl to execute all the existing commands, even some that were impossible until now like create_room_with_opts
and set_vcard2_multi
.
List elements are separated with ,
and tuple elements are separated with :
.
Relevant commands:
– add_rosteritem
– create_room_with_opts
– oauth_issue_token
– send_direct_invitation
– set_vcard2_multi
– srg_create
– subscribe_room
– subscribe_room_many
Some example uses:
ejabberdctl add_rosteritem user1 localhost testuser7 localhost NickUser77l gr1,gr2,gr3 both
ejabberdctl create_room_with_opts room1 conference.localhost localhost public:false,persistent:true
ejabberdctl subscribe_room_many user1@localhost:User1,admin@localhost:Admin room1@conference.localhost urn:xmpp:mucsub:nodes:messages,u
ejabberdctl list results
Until now, ejabberdctl returned list elements separated with ;
. Now in API version 1 list elements are separated with ,
.
For example, in ejabberd 23.10:
$ ejabberdctl get_roster admin localhost
jan@localhost jan none subscribe group1;group2
tom@localhost tom none subscribe group3
Since this ejabberd release, using API version 1:
$ ejabberdctl get_roster admin localhost
jan@localhost jan none subscribe group1,group2
tom@localhost tom none subscribe group3
it is still possible to get the results in the old syntax, using API version 0:
$ ejabberdctl --version 0 get_roster admin localhost
jan@localhost jan none subscribe group1;group2
tom@localhost tom none subscribe group3
ejabberdctl help improved
ejabberd supports around 200 administrative commands, and probably you consult them in the ejabberd Docs -> API Reference page, where all the commands documentation is perfectly displayed…
The ejabberdctl
command-line script already allowed to consult the commands documentation, consulting in real-time your ejabberd server to show you exactly the commands that are available. But it lacked some details about the commands. That has been improved, and now ejabberdctl
shows all the information, including arguments description, examples and version notes.
For example, the connected_users_vhost
command documentation as seen in the ejabberd Docs site is equivalently visible using ejabberdctl
:
$ ejabberdctl help connected_users_vhost
Command Name: connected_users_vhost
Arguments: host::binary : Server name
Result: connected_users_vhost::[ sessions::string ]
Example: ejabberdctl connected_users_vhost "myexample.com"
user1@myserver.com/tka
user2@localhost/tka
Tags: session
Module: mod_admin_extra
Description: Get the list of established sessions in a vhost
Experimental support for Erlang/OTP 27
Erlang/OTP 27.0-rc1 was recently released, and ejabberd can be compiled with it. If you are developing or experimenting with ejabberd, it would be great if you can use Erlang/OTP 27 and report any problems you find. For production servers, it’s recommended to stick with Erlang/OTP 26.2 or any previous version.
In this sense, the rebar
and rebar3
binaries included with ejabberd are also updated: now they support from Erlang 24 to Erlang 27. If you want to use older Erlang versions from 20 to 23, there are compatible binaries available in git: rebar from ejabberd 21.12 and rebar3 from ejabberd 21.12.
Of course, if you have rebar
or rebar3
already installed in your system, it’s preferable if you use those ones, because probably they will be perfectly compatible with whatever erlang version you have installed.
Installers and ejabberd
container image
The binary installers now include the recent and stable Erlang/OTP 26.2.2 and Elixir 1.16.1. Many other dependencies were updated in the installers, the most notable is OpenSSL that has jumped to version 3.2.1.
The ejabberd
container image and the ecs
container image have gotten all those version updates, and also Alpine is updated to 3.19.
By the way, this container image already had support to run commands when the container starts… And now you can setup the commands to allow them fail, by prepending the character !
.
Summary of compilation methods
When compiling ejabberd from source code, you may have noticed there are a lot of possibilities. Let’s take an overview before digging in the new improvements:
- Tools to manage the dependencies and compilation:
- Rebar: it is nowadays very obsolete, but still does the job of compiling ejabberd
- Rebar3: the successor of Rebar, with many improvements and plugins, supports hex.pm and Elixir compilation
- Mix: included with the Elixir programming language, supports hex.pm, and erlang compilation
- Installation methods:
make install
: copies the files to the system
make prod
: prepares a self-contained OTP production release in _build/prod/
, and generates a tar.gz
file. This was previously named make rel
make dev
: prepares quickly an OTP development release in _build/dev/
make relive
: prepares the barely minimum in _build/relive/
to run ejabberd and starts it
- Start scripts and alternatives:
ejabberdctl
with erlang shell: start
/foreground
/live
ejabberdctl
with elixir shell: iexlive
ejabberd
console
/start
(this script is generated by rebar3 or mix, and does not support ejabberdctl configurable options)
For example:
– the CI
dynamic tests use rebar3
, and Runtime
tries to test all the possible combinations
– ejabberd binary installers are built using: mix + make prod
– container images are built using: mix + make prod
too, and started with ejabberdctl foreground
Several combinations didn’t work correctly until now and have been fixed, for example:
– mix + make relive
– mix + make prod/dev + ejabberdctl iexlive
– mix + make install + ejabberdctl start/foregorund/live
– make uninstall
buggy has an experimental alternative: make uninstall-rel
– rebar + make prod
with Erlang 26
Use Mix or Rebar3 by default instead of Rebar to compile ejabberd
ejabberd uses Rebar to manage dependencies and compilation since ejabberd 13.10 4d8f770. However, that tool is obsolete and unmaintained since years ago, because there is a complete replacement:
Rebar3 is supported by ejabberd since 20.12 0fc1aea. Among other benefits, this allows to download dependencies from hex.pm and cache them in your system instead of downloading them from git every time, and allows to compile Elixir files and Elixir dependencies.
In fact, ejabberd can be compiled using mix
(a tool included with the Elixir programming language) since ejabberd 15.04 ea8db99 (with improvements in ejabberd 21.07 4c5641a)
For those reasons, the tool selection performed by ./configure
will now be:
– If --with-rebar=rebar3
but Rebar3 not found installed in the system, use the rebar3
binary included with ejabberd
– Use the program specified in option: --with-rebar=/path/to/bin
– If none is specified, use the system mix
– If Elixir not found, use the system rebar3
– If Rebar3 not found, use the rebar3
binary included with ejabberd
Removed Elixir support in Rebar
Support for Elixir 1.1 was added as a dependency in commit 01e1f67 to ejabberd 15.02. This allowed to compile Elixir files. But since Elixir 1.4.5 (released Jun 22, 2017) it isn’t possible to get Elixir as a dependency… it’s nowadays a standalone program. For that reason, support to download old Elixir 1.4.4 as a dependency has been removed.
When Elixir support is required, better simply install Elixir and use mix
as build tool:
./configure --with-rebar=mix
Or install Elixir and use the experimental Rebar3 support to compile Elixir files and dependencies:
./configure --with-rebar=rebar3 --enable-elixir
Added Elixir support in Rebar3
It is now possible to compile ejabberd using Rebar3 and support Elixir compilation. This compiles the Elixir files included in ejabberd’s lib/
path. There’s also support to get dependencies written in Elixir, and it’s possible to build OTP releases including Elixir support.
It is necessary to have Elixir installed in the system, and configure the compilation using --enable-elixir
. For example:
apt-get install erlang erlang-dev elixir
git clone https://github.com/processone/ejabberd.git ejabberd
cd ejabberd
./autogen.sh
./configure --with-rebar=rebar3 --enable-elixir
make
make dev
_build/dev/rel/ejabberd/bin/ejabberdctl iexlive
Elixir versions supported
Elixir 1.10.3 is the minimum supported, but:
– Elixir 1.10.3 or higher is required to build an OTP release with make prod
or make dev
– Elixir 1.11.4 or higher is required to build an OTP release if using Erlang/OTP 24 or higher
– Elixir 1.11.0 or higher is required to use make relive
– Elixir 1.13.4 with Erlang/OTP 23.0 are the lowest versions tested by Runtime
For all those reasons, if you want to use Elixir, it is highly recommended to use Elixir 1.13.4 or higher with Erlang/OTP 23.0 or higher.
make rel
is renamed to make prod
When ejabberd started to use Rebar2 build tool, that tool could create an OTP release, and the target in Makefile.in
was conveniently named make rel
.
However, newer tools like Rebar3 and Elixir’s Mix support creating different types of releases: production, development, … In this sense, our make rel
target is nowadays more properly named make prod
.
For backwards compatibility, make rel
redirects to make prod
.
New make install-rel
and make uninstall-rel
This is an alternative method to install ejabberd in the system, based in the OTP release process. It should produce exactly the same results than the existing make install
.
The benefits of make install-rel
over the existing method:
– this uses OTP release code from rebar/rebar3/mix, and consequently requires less code in our Makefile.in
– make uninstall-rel
correctly deletes all the library files
This is still experimental, and it would be great if you are able to test it and report any problem; eventually this method could replace the existing one.
Just for curiosity:
– ejabberd 13.03-beta1 got support for make uninstall
was added
– ejabberd 13.10 introduced Rebar build tool and code got more modular
– ejabberd 15.10 started to use the OTP directory structure for ‘make install’, and this broke make uninstall
Acknowledgments
We would like to thank the contributions to the source code, documentation, and translation provided for this release by:
And also to all the people contributing in the ejabberd chatroom, issue tracker…
Improvements in ejabberd Business Edition
Customers of the ejabberd Business Edition, in addition to all those improvements and bugfixes, also get:
Push
- Fix clock issue when signing Apple push JWT tokens
- Share Apple push JWT tokens between nodes in cluster
- Increase allowed certificates chain depth in GCM requests
- Use
x:oob
data as source for image delivered in pushes
- Process only https urls in oob as images in pushes
- Fix jid in disable push iq generated by GCM and Webhook service
- Add better logging for
TooManyProviderTokenUpdated
error
- Make
get_push_logs
command generate better error if mod_push_logger
not available
- Add command
get_push_logs
that can be used to retrieve info about recent pushes and errors reported by push services
- Add support for webpush protocol for sending pushes to safari/chrome/firefox browsers
MAM
- Expand
mod_mam_http_access
API to also accept range of messages
MUC
- Update
mod_muc_state_query
to fix subject_author
room state field
- Fix encoding of config
xdata
in mod_muc_state_query
PubSub
- Allow pubsub node owner to overwrite items published by other persons (p1db)
ChangeLog
This is a more detailed list of changes in this ejabberd release:
Core
- Added Matrix gateway in
mod_matrix_gw
- Support SASL2 and Bind2
- Support tls-server-end-point channel binding and sasl2 codec
- Support tls-exporter channel binding
- Support XEP-0474: SASL SCRAM Downgrade Protection
- Fix presenting features and returning results of inline bind2 elements
disable_sasl_scram_downgrade_protection
: New option to disable XEP-0474
negotiation_timeout
: Increase default value from 30s to 2m
- mod_carboncopy: Teach how to interact with bind2 inline requests
Other
- ejabberdctl: Fix startup problem when having set
EJABBERD_OPTS
and logger options
- ejabberdctl: Set EJABBERD_OPTS back to
""
, and use previous flags as example
- eldap: Change logic for
eldap tls_verify=soft
and false
- eldap: Don’t set
fail_if_no_peer_cert
for eldap ssl client connections
- Ignore hints when checking for chat states
- mod_mam: Support XEP-0424 Message Retraction
- mod_mam: Fix XEP-0425: Message Moderation with SQL storage
- mod_ping: Support XEP-0198 pings when stream management is enabled
- mod_pubsub: Normalize pubsub
max_items
node options on read
- mod_pubsub: PEP nodetree: Fix reversed logic in node fixup function
- mod_pubsub: Only care about PEP bookmarks options when creating node from scratch
SQL
- MySQL: Support
sha256_password
auth plugin
- ejabberd_sql_schema: Use the first unique index as a primary key
- Update SQL schema files for MAM’s XEP-0424
- New option
sql_flags
: right now only useful to enable mysql_alternative_upsert
Installers and Container
- Container: Add ability to ignore failures in execution of
CTL_ON_*
commands
- Container: Update to Erlang/OTP 26.2, Elixir 1.16.1 and Alpine 3.19
- Container: Update this custom ejabberdctl to match the main one
- make-binaries: Bump OpenSSL 3.2.1, Erlang/OTP 26.2.2, Elixir 1.16.1
- make-binaries: Bump many dependency versions
Commands API
print_sql_schema
: New command available in ejabberdctl command-line script
- ejabberdctl: Rework temporary node name generation
- ejabberdctl: Print argument description, examples and note in help
- ejabberdctl: Document exclusive ejabberdctl commands like all the others
- Commands: Add a new
muc_sub
tag to all the relevant commands
- Commands: Improve syntax of many commands documentation
- Commands: Use list arguments in many commands that used separators
- Commands:
set_presence
: switch priority argument from string to integer
- ejabberd_commands: Add the command API version as a tag
vX
- ejabberd_ctl: Add support for list and tuple arguments
- ejabberd_xmlrpc: Fix support for restuple error response
- mod_http_api: When no specific API version is requested, use the latest
Compilation with Rebar3/Elixir/Mix
- Fix compilation with Erlang/OTP 27: don’t use the reserved word ‘maybe’
- configure: Fix explanation of
--enable-group
option (#4135)
- Add observer and runtime_tools in releases when
--enable-tools
- Update “make translations” to reduce build requirements
- Use Luerl 1.0 for Erlang 20, 1.1.1 for 21-26, and temporary fork for 27
- Makefile: Add
install-rel
and uninstall-rel
- Makefile: Rename
make rel
to make prod
- Makefile: Update
make edoc
to use ExDoc, requires mix
- Makefile: No need to use
escript
to run rebar|rebar3|mix
- configure: If
--with-rebar=rebar3
but rebar3 not system-installed, use local one
- configure: Use Mix or Rebar3 by default instead of Rebar2 to compile ejabberd
- ejabberdctl: Detect problem running iex or etop and show explanation
- Rebar3: Include Elixir files when making a release
- Rebar3: Workaround to fix protocol consolidation
- Rebar3: Add support to compile Elixir dependencies
- Rebar3: Compile explicitly our Elixir files when
--enable-elixir
- Rebar3: Provide proper path to
iex
- Rebar/Rebar3: Update binaries to work with Erlang/OTP 24-27
- Rebar/Rebar3: Remove Elixir as a rebar dependency
- Rebar3/Mix: If
dev
profile/environment, enable tools automatically
- Elixir: Fix compiling ejabberd as a dependency (#4128)
- Elixir: Fix ejabberdctl start/live when installed
- Elixir: Fix:
FORMATTER ERROR: bad return value
(#4087)
- Elixir: Fix: Couldn’t find file
Elixir Hex API
- Mix: Enable stun by default when
vars.config
not found
- Mix: New option
vars_config_path
to set path to vars.config
(#4128)
- Mix: Fix ejabberdctl iexlive problem locating iex in an OTP release
Full Changelog
https://github.com/processone/ejabberd/compare/23.10…24.02
ejabberd 24.02 download & feedback
As usual, the release is tagged in the Git source code repository on GitHub.
The source package and installers are available in ejabberd Downloads page. To check the *.asc
signature files, see How to verify ProcessOne downloads integrity.
For convenience, there are alternative download locations like the ejabberd DEB/RPM Packages Repository and the GitHub Release / Tags.
The ecs
container image is available in docker.io/ejabberd/ecs and ghcr.io/processone/ecs. The alternative ejabberd
container image is available in ghcr.io/processone/ejabberd.
If you consider that you’ve found a bug, please search or fill a bug report on GitHub Issues.
The post
ejabberd 24.02 first appeared on
ProcessOne.